A capability is a bunch of explanations that takes input, does some particular calculation, and produces yield. The thought is to put some normally or more than once taken care of errands together to make a capability so that as opposed to composing a similar code over and over for various data sources, we can call this capability.
In basic terms, a capability is a block of code that runs just when it is called.
Syntax:
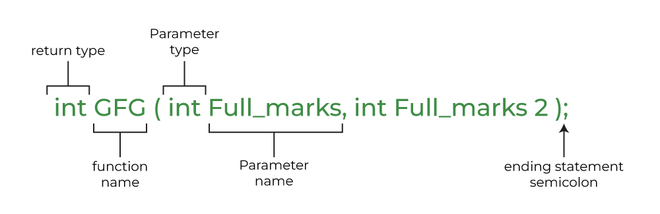
Syntax of Function
Example:
- C++
Output
m is 20
For what reason Do We Want Capabilities?
Capabilities help us in diminishing code overt repetitiveness. On the off chance that usefulness is performed at various spots in programming, as opposed to composing a similar code, over and over, we make a capability and call it all over the place. This additionally assists in support as we with making changes in just a single spot in the event that we make changes to the usefulness in future.
Capabilities make code particular. Consider a major record having many lines of code. It turns out to be truly easy to peruse and utilize the code, in the event that the code is partitioned into capabilities.
Capabilities give deliberation. For instance, we can utilize library capabilities without stressing over their inward work.
Capability Announcement
A capability statement educates the compiler concerning the quantity of boundaries, information kinds of boundaries, and returns sort of capability. Composing boundary names in the capability statement is discretionary yet placing them in the definition is essential. The following is an illustration of capability statements. (boundary names are absent in the underneath statements)
Capability Announcement in C++
Capability Announcement
Model:
C++
No comments:
Post a Comment